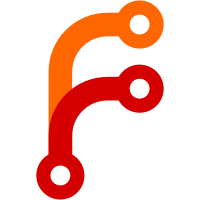
Pull AFS fixes from David Howells: "Here's a set of patches that fix a number of bugs in the in-kernel AFS client, including: - Fix directory locking to not use individual page locks for directory reading/scanning but rather to use a semaphore on the afs_vnode struct as the directory contents must be read in a single blob and data from different reads must not be mixed as the entire contents may be shuffled about between reads. - Fix address list parsing to handle port specifiers correctly. - Only give up callback records on a server if we actually talked to that server (we might not be able to access a server). - Fix some callback handling bugs, including refcounting, whole-volume callbacks and when callbacks actually get broken in response to a CB.CallBack op. - Fix some server/address rotation bugs, including giving up if we can't probe a server; giving up if a server says it doesn't have a volume, but there are more servers to try. - Fix the decoding of fetched statuses to be OpenAFS compatible. - Fix the handling of server lookups in Cache Manager ops (such as CB.InitCallBackState3) to use a UUID if possible and to handle no server being found. - Fix a bug in server lookup where not all addresses are compared. - Fix the non-encryption of calls that prevents some servers from being accessed (this also requires an AF_RXRPC patch that has already gone in through the net tree). There's also a patch that adds tracepoints to log Cache Manager ops that don't find a matching server, either by UUID or by address" * tag 'afs-fixes-20180514' of git://git.kernel.org/pub/scm/linux/kernel/git/dhowells/linux-fs: afs: Fix the non-encryption of calls afs: Fix CB.CallBack handling afs: Fix whole-volume callback handling afs: Fix afs_find_server search loop afs: Fix the handling of an unfound server in CM operations afs: Add a tracepoint to record callbacks from unlisted servers afs: Fix the handling of CB.InitCallBackState3 to find the server by UUID afs: Fix VNOVOL handling in address rotation afs: Fix AFSFetchStatus decoder to provide OpenAFS compatibility afs: Fix server rotation's handling of fileserver probe failure afs: Fix refcounting in callback registration afs: Fix giving up callbacks on server destruction afs: Fix address list parsing afs: Fix directory page locking
85 lines
1.6 KiB
C
85 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Simple pointer stack
|
|
*
|
|
* (c) 2010 Arnaldo Carvalho de Melo <acme@redhat.com>
|
|
*/
|
|
|
|
#include "util.h"
|
|
#include "pstack.h"
|
|
#include "debug.h"
|
|
#include <linux/kernel.h>
|
|
#include <stdlib.h>
|
|
|
|
struct pstack {
|
|
unsigned short top;
|
|
unsigned short max_nr_entries;
|
|
void *entries[0];
|
|
};
|
|
|
|
struct pstack *pstack__new(unsigned short max_nr_entries)
|
|
{
|
|
struct pstack *pstack = zalloc((sizeof(*pstack) +
|
|
max_nr_entries * sizeof(void *)));
|
|
if (pstack != NULL)
|
|
pstack->max_nr_entries = max_nr_entries;
|
|
return pstack;
|
|
}
|
|
|
|
void pstack__delete(struct pstack *pstack)
|
|
{
|
|
free(pstack);
|
|
}
|
|
|
|
bool pstack__empty(const struct pstack *pstack)
|
|
{
|
|
return pstack->top == 0;
|
|
}
|
|
|
|
void pstack__remove(struct pstack *pstack, void *key)
|
|
{
|
|
unsigned short i = pstack->top, last_index = pstack->top - 1;
|
|
|
|
while (i-- != 0) {
|
|
if (pstack->entries[i] == key) {
|
|
if (i < last_index)
|
|
memmove(pstack->entries + i,
|
|
pstack->entries + i + 1,
|
|
(last_index - i) * sizeof(void *));
|
|
--pstack->top;
|
|
return;
|
|
}
|
|
}
|
|
pr_err("%s: %p not on the pstack!\n", __func__, key);
|
|
}
|
|
|
|
void pstack__push(struct pstack *pstack, void *key)
|
|
{
|
|
if (pstack->top == pstack->max_nr_entries) {
|
|
pr_err("%s: top=%d, overflow!\n", __func__, pstack->top);
|
|
return;
|
|
}
|
|
pstack->entries[pstack->top++] = key;
|
|
}
|
|
|
|
void *pstack__pop(struct pstack *pstack)
|
|
{
|
|
void *ret;
|
|
|
|
if (pstack->top == 0) {
|
|
pr_err("%s: underflow!\n", __func__);
|
|
return NULL;
|
|
}
|
|
|
|
ret = pstack->entries[--pstack->top];
|
|
pstack->entries[pstack->top] = NULL;
|
|
return ret;
|
|
}
|
|
|
|
void *pstack__peek(struct pstack *pstack)
|
|
{
|
|
if (pstack->top == 0)
|
|
return NULL;
|
|
return pstack->entries[pstack->top - 1];
|
|
}
|